plot_contour_labels
#
This notebook is a simple example of the GeoCAT-viz function plot_contour_labels.
# Import packages:
import xarray as xr
import matplotlib.pyplot as plt
import cartopy.crs as ccrs
import cartopy.feature as cfeature
import numpy as np
import geocat.datafiles as gdf
import geocat.viz as gv
# Read in data:
# Open a netCDF data file using xarray default engine and
# load the data into xarrays
ds = xr.open_dataset(gdf.get("netcdf_files/slp.1963.nc"), decode_times=False)
# Get data from the 24th timestep
pressure = ds.slp[24, :, :]
# Translate short values to float values
pressure = pressure.astype('float64')
# Convert Pa to hPa data
pressure = pressure * 0.01
# Fix the artifact of not-shown-data around 0 and 360-degree longitudes
wrap_pressure = gv.xr_add_cyclic_longitudes(pressure, "lon")
Downloading file 'netcdf_files/slp.1963.nc' from 'https://github.com/NCAR/GeoCAT-datafiles/raw/main/netcdf_files/slp.1963.nc' to '/home/docs/.cache/geocat'.
/home/docs/checkouts/readthedocs.org/user_builds/geocat-viz/conda/stable/lib/python3.12/site-packages/xarray/coding/times.py:170: SerializationWarning: Ambiguous reference date string: 1-1-1 00:00:0.0. The first value is assumed to be the year hence will be padded with zeros to remove the ambiguity (the padded reference date string is: 0001-1-1 00:00:0.0). To remove this message, remove the ambiguity by padding your reference date strings with zeros.
warnings.warn(warning_msg, SerializationWarning)
# Create plot
# Set figure size
fig = plt.figure(figsize=(8, 8))
# Set global axes with an orthographic projection
proj = ccrs.Orthographic(central_longitude=270, central_latitude=45)
ax = plt.axes(projection=proj)
ax.set_global()
# Plot contour data
p = wrap_pressure.plot.contour(ax=ax,
transform=ccrs.PlateCarree(),
linewidths=0.5,
cmap='black',
add_labels=False)
contour_label_locations = [(176.4, 34.63), (-150.46, 42.44), (-142.16, 28.5),
(-92.49, 25.64), (-156.05, 84.47), (-17.83, 82.52),
(-76.3, 41.99), (-48.89, 41.45), (-33.43, 37.55)]
# Plot Clabels
gv.plot_contour_labels(ax,
p,
ccrs.Geodetic(),
proj,
clabel_locations=contour_label_locations)
# Make layout tight
plt.tight_layout()
plt.show();
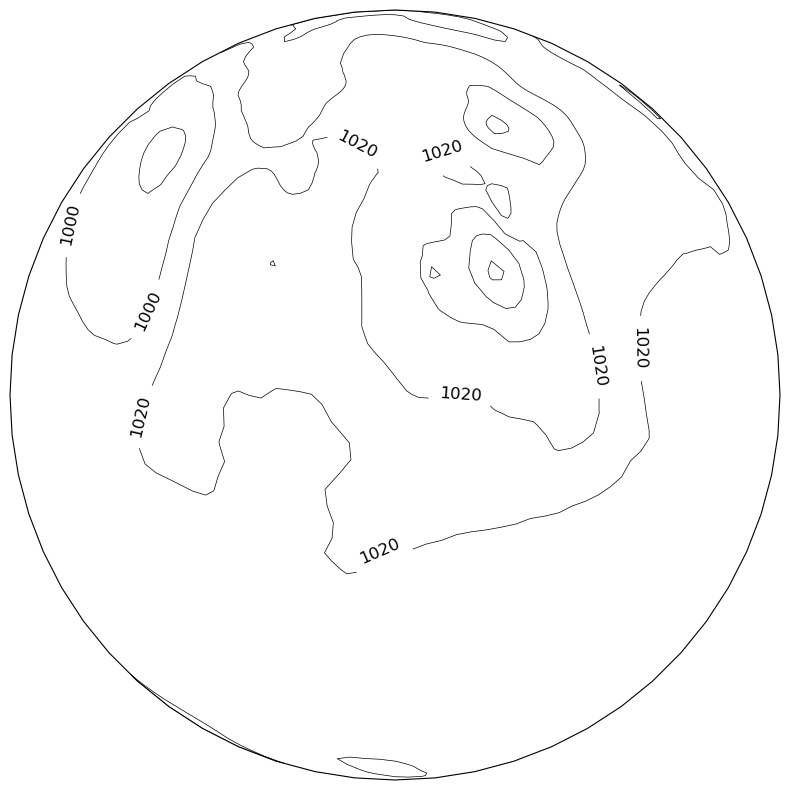